Home › Forums › MagCAD Support › HDL Models › unable to get the output waveform › Reply To: unable to get the output waveform
February 21, 2018 at 2:24 am
#1290
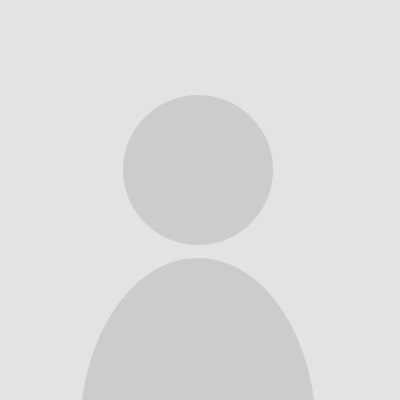
Participant
Sir,
Here I am giving the “definition.vhd” . Kindly try my layout and let me know why am I unable to get the output.
--------------------------------------------------------------------------------
-- Definitions and parameters automatically generate by MagCAD
--
-- Please use MagCAD to change technological parameters
--
--------------------------------------------------------------------------------
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
use ieee.math_real.all;
package definitions_pnml is
--circuit parameters
constant longest_path : integer := 5; --number of magnet crossed by the longest path
--fields constants
constant H_pulse : real := 560.0; --field pulse amplitude [Oe]
constant H_clock : real := 560.0; --clocking field amplitude[Oe]
constant H_int : real := 190.0; --intrinsic pinning field[Oe]
--time constants
constant T_prop : real := 1.1e-08; --Original value 1.05396e-08 effective clock pulse [s]
constant T_nuc : real := 5.6e-07; --Original value 5.5752e-07 nucleation time [s]
--Probability extremes
constant P_nuc_critical : real := 0.95; --critical nucleation probability
constant P_prev_critical : real := 0.05; --critical nucleation prevent probability
--base components constants
constant dw_length : real := 1.0e-07; --grid size [meters]
constant dw_width : real := 2.0e-08; --width of the domainwall [meters]
constant v_00 : real := 1.14e+06; --numerical prefactor in depinning regime [m/s]
constant E_pin : real := 12.1; --pinning energy barrier (incluedes the K_bT constant)
constant v_0 : real := 31.9; --numerical prefactor in flow regime [m/s]
constant u_w : real := 0.042; --domain wall mobility [m/sOe]
constant C_inv : real := 153.0; --Coupling field strength [Oe]
constant C_mv : real := 48.0; --coupling field
constant f_0 : real := 2.0e+09; --Attempt frequency [Hz]
constant M_co : real := 1.4e+06; --Saturation magnetization Co
constant t_Co : real := 3.2e-09; --Co thickness [m]
constant t_stack : real := 6.2e-09; --stack thickness [m]
constant K_eff : real := 2.0e+05; --effective anisotropy [J/m^3]
constant u_0 : real := 1.256e-6; --permeability constant
constant g_FIB : real := 0.1416; --fib irradiation factor
constant V_ANC : real := 1.68e-23; --volume of the ANC
constant K_B : real := 1.3807e-23; --Boltzmann constant
constant T : real := 293.0; --temperature in Kelvin
--notch component constants
constant A : real := 1.3e-11; --exchange stiffness
constant apex : real :=(51.5* MATH_PI)/180.0; --apex angle
constant h : real := 5.4e-08; --notch width
constant V_a : real := 1.26e-23; --Activation volume
constant P_dep : real := 0.98; --Used to find the T_sync (1.0 - EXP(-T_sync/Tau_eff_notch) )
--domainwall via constants
constant C_via : real := 75.0; --coupling field for the via
--Formula for the parameters
constant M_s : real := M_Co * (t_Co/T_stack); --saturation magnetization
constant K_anc : real := g_FIB * K_eff; --ANC anisotropy
constant H_0 : real := ((2.0 * K_anc)/(u_0*M_s))*0.01256; --coercive field at zero temperature
constant E_0 : real := (K_anc * V_ANC)/(K_B*T); --energy barrier at 0 field (incluedes the K_bT constant)
constant T_eff : real := T_prop + T_nuc;
constant T_rise : real := T_eff / 4.0;
constant T_clock : real := T_eff + T_rise; --Effective pulse time
--NOTCH parameters formulas
constant d_W : real := MATH_PI*SQRT(A/K_eff); --characteristic DW width
constant o_W : real := 4.0 * SQRT(A*K_eff); --DW energy density
constant H_depin : real := H_int + (o_W * SIN(apex))/(2.0*M_s*(h + 0.5*d_W*SIN(apex)))*10000.0; --Depinning Field
constant H_sync : real := H_depin - H_pulse + 1.0; --In plane field
constant H_eff_notch : real := H_pulse + H_sync; --Effective field to depin a notch
constant T_depin : real := (1.0/f_0) * EXP((M_s*V_a*((H_depin - H_eff_notch)/10000.0))/(K_B*T)); --Depinning time
constant E_barrier_notch : real := (M_s*V_a*(H_depin - H_eff_notch))/10000.0; --Energy barrier
constant Tau_eff_notch : real := (1.0/f_0) * EXP(E_barrier_notch/(K_b*T)); --Effective time to depin a notch
constant t_sync : real := -Tau_eff_notch*LOG(1.0 - P_dep); --In plane pulse time
--generic model constants
--Set here the number of parameters that you want to analyse: e.g. the critical path.
constant NParameters : integer := 3; --number of model parameters
constant par_TIME : integer := 0; --position of propagation time in PARAM_DATA. Here each component add its delay
constant par_TIME_NUC : integer := 1;
constant par_CR_TIME : integer := 2; --position of critical propagation time in PARAM_DATA. Here is memorized the current critical path.
--data type
--vector including parameters of the model defined in the generic constants
type param_data is array(0 to NParameters -1) of real;
type param_data_vector is array(integer range <>) of param_data;
--DELCARATIONS
--functions declarations
function propagation_time return real;
function propagation_length return real;
function t_nuc_via return real;
--procedure to determine the velocity_DW according to the H_pulse, so to the regime
procedure velocity_dw (v_DW : out real);
--procedure to compute the probability of nucleation. It uses the current state of the circuit to compute the probaility to nucleate or to ramin in the current state
procedure probability_nuc (signal input_state: in std_logic; signal current_state: in std_logic; P_nuc: out real; P_prev : out real);
procedure probability_mv (signal input_A: in std_logic; signal input_B: in std_logic; signal input_C: in std_logic; signal current_state: in std_logic; P_nuc: out real; P_prev : out real);
procedure probability_via(signal input_state: in std_logic; signal current_state: in std_logic; P_nuc: out real; P_prev : out real);
procedure probability_mv5 (signal input_A: in std_logic; signal input_B: in std_logic; signal input_C: in std_logic; signal input_D: in std_logic; signal input_E: in std_logic; signal current_state: in std_logic; P_nuc: out real; P_prev : out real);
--procedure to compute the critical path.
procedure critical_path_computation (signal param_data_in : param_data; critical_path : out real);
procedure critical_path_computation_mv (signal param_data_in_A : param_data; signal param_data_in_B : param_data; signal param_data_in_C : param_data; critical_path : out real);
procedure critical_path_computation_mv5 (signal param_data_in_A : param_data; signal param_data_in_B : param_data; signal param_data_in_C : param_data; signal param_data_in_D : param_data; signal param_data_in_E : param_data; critical_path : out real);
--function to compute the minimum nucleation time
function minimum_nuc_time return real;
end definitions_pnml;
----------------------------------------- BODY -----------------------------------------
package body definitions_pnml is
procedure velocity_DW(v_DW : out real) is
begin
if (H_pulse < H_int) then
v_DW := 1.0;
elsif (H_pulse > H_int and H_pulse < 330.0) then
v_DW := v_00 * EXP(E_pin*(H_int/H_pulse)**0.25);
elsif (H_pulse >= 330.0 and H_pulse < 750.0) then
v_DW := v_0 + u_w*(H_pulse - H_int);
elsif (H_pulse >= 750.0) then
v_DW := 57.0;
end if;
end velocity_DW;
function propagation_time return real is
variable result : real;
variable velocity : real;
begin
velocity_DW(velocity);
result := dw_length / velocity;
return result;
end;
function propagation_length return real is
variable result : real;
variable velocity : real;
begin
velocity_DW(velocity);
result := velocity * T_eff;
return result;
end;
function t_nuc_via return real is
variable result : real;
variable H_eff_via, Tau_eff_via : real;
begin
H_eff_via := H_pulse + C_via;
Tau_eff_via := (1.0/f_0) * EXP(E_0*(1.0-(H_eff_via/H_0))**2.0);
result := -Tau_eff_via*LOG(1.0 - P_nuc_critical);
return result;
end;
function minimum_nuc_time return real is
variable result : real;
variable H_eff_critical_mv, Tau_eff_critical_mv, T_nuc_min_mv : real;
variable H_eff_critical_nc, Tau_eff_critical_nc, T_nuc_min_nc : real;
variable H_eff_critical_via, Tau_eff_critical_via, T_nuc_min_via : real;
begin
H_eff_critical_mv := H_pulse + C_mv;
Tau_eff_critical_mv := (1.0/f_0) * EXP(E_0*(1.0-(H_eff_critical_mv/H_0))**2.0);
T_nuc_min_mv := -Tau_eff_critical_mv*LOG(1.0 - P_nuc_critical);
H_eff_critical_nc := H_pulse + C_inv;
Tau_eff_critical_nc := (1.0/f_0) * EXP(E_0*(1.0-(H_eff_critical_nc/H_0))**2.0);
T_nuc_min_nc := -Tau_eff_critical_nc*LOG(1.0 - P_nuc_critical);
H_eff_critical_via := H_pulse + C_via;
Tau_eff_critical_via := (1.0/f_0) * EXP(E_0*(1.0-(H_eff_critical_via/H_0))**2.0);
T_nuc_min_via := -Tau_eff_critical_via*LOG(1.0 - P_nuc_critical);
if T_nuc_min_mv > T_nuc_min_nc and T_nuc_min_mv > T_nuc_min_via then
result := T_nuc_min_mv;
elsif T_nuc_min_nc > T_nuc_min_mv and T_nuc_min_nc > T_nuc_min_via then
result := T_nuc_min_nc;
elsif T_nuc_min_via > T_nuc_min_mv and T_nuc_min_via > T_nuc_min_nc then
result := T_nuc_min_via;
end if;
return result;
end;
procedure probability_nuc (signal input_state: in std_logic; signal current_state: in std_logic; P_nuc: out real; P_prev : out real) is
variable M : real;
variable C_eff : real;
variable H_eff : real;
variable Tau_eff : real;
variable Tau_prev : real;
begin
if current_state /= 'U' then
if input_state = current_state then
M := -1.0;
elsif input_state /= current_state then
M := 1.0;
end if;
else
M := -1.0;
end if;
C_eff := C_inv*M; --Effective coupling
H_eff := H_clock - C_eff; --Effective field
Tau_eff :=(1.0/f_0) * EXP(E_0*(1.0-(H_eff/H_0))**2.0); --switching time, reverse of switching rate
P_nuc := 1.0 - EXP(-(T_nuc/Tau_eff)); --Nucleation probability
Tau_prev :=(1.0/f_0) * EXP(E_0*(1.0-((H_pulse - C_inv)/H_0))**2.0); --switching time, reverse of switching rate in case of antiparallel coupling
P_prev := 1.0 - EXP(-(T_eff/Tau_prev)); --prevent Nucleation probability
end probability_nuc;
procedure probability_mv (signal input_A: in std_logic; signal input_B: in std_logic; signal input_C: in std_logic; signal current_state: in std_logic; P_nuc: out real; P_prev : out real) is
variable M0, M1, M2 : real;
variable C_eff : real;
variable H_eff : real;
variable Tau_eff : real;
variable Tau_prev : real;
begin
if current_state /= 'U' then
if input_A = current_state then
M0 := -1.0;
elsif input_A /= current_state then
M0 := 1.0;
end if;
if input_B = current_state then
M1 := -1.0;
elsif input_B /= current_state then
M1 := 1.0;
end if;
if input_C = current_state then
M2 := -1.0;
elsif input_C /= current_state then
M2 := 1.0;
end if;
else
M0 := -1.0;
M1 := -1.0;
M2 := -1.0;
end if;
C_eff := C_mv*M0 + C_mv*M1 + C_mv*M2; --Effective coupling
H_eff := H_clock - C_eff; --Effective field
Tau_eff :=(1.0/f_0) * EXP(E_0*(1.0-(H_eff/H_0))**2.0); --switching time, reverse of switching rate
P_nuc := 1.0 - EXP(-(T_nuc/Tau_eff)); --Nucleation probability
--worst case, so when the coupling field reduces less the H_pulse
Tau_prev :=(1.0/f_0) * EXP(E_0*(1.0-((H_pulse - C_mv)/H_0))**2.0); --switching time, reverse of switching rate in case of antiparallel coupling
P_prev := 1.0 - EXP(-(T_eff/Tau_prev)); --prevent Nucleation probability
end probability_mv;
procedure probability_via (signal input_state: in std_logic; signal current_state: in std_logic; P_nuc: out real; P_prev : out real) is
variable M : real;
variable C_eff : real;
variable H_eff : real;
variable Tau_eff : real;
variable Tau_prev : real;
begin
if current_state /= 'U' then
if input_state = current_state then
M := -1.0;
elsif input_state /= current_state then
M := 1.0;
end if;
else
M := -1.0;
end if;
C_eff := C_via*M; --Effective coupling
H_eff := H_clock - C_eff; --Effective field
Tau_eff :=(1.0/f_0) * EXP(E_0*(1.0-(H_eff/H_0))**2.0); --switching time, reverse of switching rate
P_nuc := 1.0 - EXP(-(T_nuc/Tau_eff)); --Nucleation probability
Tau_prev :=(1.0/f_0) * EXP(E_0*(1.0-((H_pulse - C_via)/H_0))**2.0); --switching time, reverse of switching rate in case of antiparallel coupling
P_prev := 1.0 - EXP(-(T_eff/Tau_prev)); --prevent Nucleation probability
end probability_via;
procedure critical_path_computation (signal param_data_in : param_data; critical_path : out real) is
variable path : real;
variable current_critical_path : real;
begin
path := param_data_in(par_TIME);
current_critical_path := param_data_in(par_CR_TIME);
if path > current_critical_path then
critical_path := path;
else
critical_path := current_critical_path;
end if;
end critical_path_computation;
procedure critical_path_computation_mv (signal param_data_in_A : param_data; signal param_data_in_B : param_data; signal param_data_in_C : param_data; critical_path : out real) is
variable path : real;
variable current_critical_path : real;
begin
--find the critical path from the inputs
if (param_data_in_A(par_TIME) >= param_data_in_B(par_TIME)) and (param_data_in_A(par_TIME) >= param_data_in_C(par_TIME)) then
path := param_data_in_A(par_TIME);
elsif param_data_in_B(par_TIME) >= param_data_in_A(par_TIME) and param_data_in_B(par_TIME) >= param_data_in_C(par_TIME) then
path := param_data_in_B(par_TIME);
elsif param_data_in_C(par_TIME) >= param_data_in_A(par_TIME) and param_data_in_C(par_TIME) >= param_data_in_B(par_TIME) then
path := param_data_in_C(par_TIME);
end if;
if (param_data_in_A(par_CR_TIME) >= param_data_in_B(par_CR_TIME)) and (param_data_in_A(par_CR_TIME) >= param_data_in_C(par_CR_TIME)) then
current_critical_path := param_data_in_A(par_CR_TIME);
elsif param_data_in_B(par_CR_TIME) >= param_data_in_A(par_CR_TIME) and param_data_in_B(par_CR_TIME) >= param_data_in_C(par_CR_TIME) then
current_critical_path := param_data_in_B(par_CR_TIME);
elsif param_data_in_C(par_CR_TIME) >= param_data_in_A(par_CR_TIME) and param_data_in_C(par_CR_TIME) >= param_data_in_B(par_CR_TIME) then
current_critical_path := param_data_in_C(par_CR_TIME);
end if;
if path > current_critical_path then
critical_path := path;
else
critical_path := current_critical_path;
end if;
end critical_path_computation_mv;
procedure probability_mv5 (signal input_A: in std_logic; signal input_B: in std_logic; signal input_C: in std_logic; signal input_D: in std_logic; signal input_E: in std_logic; signal current_state: in std_logic; P_nuc: out real; P_prev : out real) is
variable M0, M1, M2, M3, M4 : real;
variable C_eff : real;
variable H_eff : real;
variable Tau_eff : real;
variable Tau_prev : real;
begin
if current_state /= 'U' then
if input_A = current_state then
M0 := -1.0;
elsif input_A /= current_state then
M0 := 1.0;
end if;
if input_B = current_state then
M1 := -1.0;
elsif input_B /= current_state then
M1 := 1.0;
end if;
if input_C = current_state then
M2 := -1.0;
elsif input_C /= current_state then
M2 := 1.0;
end if;
if input_D = current_state then
M3 := -1.0;
elsif input_D /= current_state then
M3 := 1.0;
end if;
if input_E = current_state then
M4 := -1.0;
elsif input_E /= current_state then
M4 := 1.0;
end if;
else
M0 := -1.0;
M1 := -1.0;
M2 := -1.0;
M3 := -1.0;
M4 := -1.0;
end if;
C_eff := C_mv*M0 + C_mv*M1 + C_mv*M2 + C_mv*M3 + C_mv*M4; --Effective coupling
H_eff := H_clock - C_eff; --Effective field
Tau_eff :=(1.0/f_0) * EXP(E_0*(1.0-(H_eff/H_0))**2.0); --switching time, reverse of switching rate
P_nuc := 1.0 - EXP(-(T_nuc/Tau_eff)); --Nucleation probability
--worst case, so when the coupling field reduces less the H_pulse
Tau_prev :=(1.0/f_0) * EXP(E_0*(1.0-((H_pulse - C_mv)/H_0))**2.0); --switching time, reverse of switching rate in case of antiparallel coupling
P_prev := 1.0 - EXP(-(T_eff/Tau_prev)); --prevent Nucleation probability
end probability_mv5;
procedure critical_path_computation_mv5 (signal param_data_in_A : param_data; signal param_data_in_B : param_data; signal param_data_in_C : param_data; signal param_data_in_D : param_data; signal param_data_in_E : param_data; critical_path : out real) is
variable path : real;
variable current_critical_path : real;
begin
--find the critical path from the inputs
if (param_data_in_A(par_TIME) >= param_data_in_B(par_TIME)) and (param_data_in_A(par_TIME) >= param_data_in_C(par_TIME)) and (param_data_in_A(par_TIME) >= param_data_in_D(par_TIME)) and (param_data_in_A(par_TIME) >= param_data_in_E(par_TIME)) then
path := param_data_in_A(par_TIME);
elsif param_data_in_B(par_TIME) >= param_data_in_A(par_TIME) and (param_data_in_B(par_TIME) >= param_data_in_C(par_TIME)) and (param_data_in_B(par_TIME) >= param_data_in_D(par_TIME)) and (param_data_in_B(par_TIME) >= param_data_in_E(par_TIME)) then
path := param_data_in_B(par_TIME);
elsif param_data_in_C(par_TIME) >= param_data_in_A(par_TIME) and (param_data_in_C(par_TIME) >= param_data_in_B(par_TIME)) and (param_data_in_C(par_TIME) >= param_data_in_D(par_TIME)) and (param_data_in_C(par_TIME) >= param_data_in_E(par_TIME)) then
path := param_data_in_C(par_TIME);
elsif (param_data_in_D(par_TIME) >= param_data_in_A(par_TIME)) and (param_data_in_D(par_TIME) >= param_data_in_B(par_TIME)) and (param_data_in_D(par_TIME) >= param_data_in_C(par_TIME)) and (param_data_in_D(par_TIME) >= param_data_in_E(par_TIME)) then
path := param_data_in_D(par_TIME);
elsif (param_data_in_E(par_TIME) >= param_data_in_A(par_TIME)) and (param_data_in_E(par_TIME) >= param_data_in_B(par_TIME)) and (param_data_in_E(par_TIME) >= param_data_in_C(par_TIME)) and (param_data_in_E(par_TIME) >= param_data_in_D(par_TIME)) then
path := param_data_in_E(par_TIME);
end if;
if (param_data_in_A(par_CR_TIME) >= param_data_in_B(par_CR_TIME)) and (param_data_in_A(par_CR_TIME) >= param_data_in_C(par_CR_TIME)) and (param_data_in_A(par_CR_TIME) >= param_data_in_D(par_CR_TIME)) and (param_data_in_A(par_CR_TIME) >= param_data_in_E(par_CR_TIME)) then
current_critical_path := param_data_in_A(par_CR_TIME);
elsif param_data_in_B(par_CR_TIME) >= param_data_in_A(par_CR_TIME) and (param_data_in_B(par_CR_TIME) >= param_data_in_C(par_CR_TIME)) and (param_data_in_B(par_CR_TIME) >= param_data_in_D(par_CR_TIME)) and (param_data_in_B(par_CR_TIME) >= param_data_in_E(par_CR_TIME)) then
current_critical_path := param_data_in_B(par_CR_TIME);
elsif param_data_in_C(par_CR_TIME) >= param_data_in_A(par_CR_TIME) and (param_data_in_C(par_CR_TIME) >= param_data_in_B(par_CR_TIME)) and (param_data_in_C(par_CR_TIME) >= param_data_in_D(par_CR_TIME)) and (param_data_in_C(par_CR_TIME) >= param_data_in_E(par_CR_TIME)) then
current_critical_path := param_data_in_C(par_CR_TIME);
elsif (param_data_in_D(par_CR_TIME) >= param_data_in_A(par_CR_TIME)) and (param_data_in_D(par_CR_TIME) >= param_data_in_B(par_CR_TIME)) and (param_data_in_D(par_CR_TIME) >= param_data_in_C(par_CR_TIME)) and (param_data_in_D(par_CR_TIME) >= param_data_in_E(par_CR_TIME)) then
current_critical_path := param_data_in_D(par_CR_TIME);
elsif (param_data_in_E(par_CR_TIME) >= param_data_in_A(par_CR_TIME)) and (param_data_in_E(par_CR_TIME) >= param_data_in_B(par_CR_TIME)) and (param_data_in_E(par_CR_TIME) >= param_data_in_C(par_CR_TIME)) and (param_data_in_E(par_CR_TIME) >= param_data_in_D(par_CR_TIME)) then
current_critical_path := param_data_in_E(par_CR_TIME);
end if;
if path > current_critical_path then
critical_path := path;
else
critical_path := current_critical_path;
end if;
end critical_path_computation_mv5;
end definitions_pnml;
-
This reply was modified 7 years, 4 months ago by
Umberto. Reason: please use the code tags to open/close a code snippet